Section 1 content
Widgets
Reusable components to speed up your development!
Alert Renders Foundation alerts
The widget Alert differs from the helper in a way that the widget renders automatically the required scripts if they are not yet loaded and also checks against Flash messages to render alerts. For those knowing Yii-Bootstrap or YiiStrap, well, this widget works exactly as the Alert widget on those extension libraries.
Heads up! Widgets in YiiFoundation have a special method to render the widgets without the need to use widget or beginWidget methods. It was inspired by Yii2's widget method.
Example
<?php use foundation\widgets\Alert;?> <?php Yii::app()->user->setFlash('success', 'A success message!');?> <?php echo foundation\widgets\Alert::display();?>
Breadcrumbs Renders, ehem, breadcrumbs
Breadcrumbs come in handy to show a navigation trail for users clicking through a site or app. They'll conform to 100% of the container width you put them in.
Example
<?php $this->widget( 'foundation\widgets\Breadcrumbs', array( 'items' => array( 'Home' => '#', 'features' => array( 'url' => '/features', 'options' => array('class' => 'unavailable') ), 'cloning' => array('options' => array('class' => 'current'), 'url' => '#') ) ) );?>
Clearing Lightbox Foundation style
Clearing makes it easy to create responsive lightboxes with any size image.
Example
echo foundation\widgets\Clearing::display( array( 'items' => array( array( 'th' => $this->baseUrl('images/demos/demo1-th.jpg'), 'img' => $this->baseUrl('images/demos/demo1.jpg'), 'caption' => 'My Caption 1' ), array( 'th' => $this->baseUrl('images/demos/demo2-th.jpg'), 'img' => $this->baseUrl('images/demos/demo2.jpg'), 'caption' => 'My Caption 2' ), array( 'th' => $this->baseUrl('images/demos/demo3-th.jpg'), 'img' => $this->baseUrl('images/demos/demo3.jpg'), 'caption' => 'My Caption 3' ), array( 'th' => $this->baseUrl('images/demos/demo4-th.jpg'), 'img' => $this->baseUrl('images/demos/demo4.jpg'), 'caption' => 'My Caption 4' ), array( 'th' => $this->baseUrl('images/demos/demo5-th.jpg'), 'img' => $this->baseUrl('images/demos/demo5.jpg'), 'caption' => 'My Caption 5' ) ) ) );
Dropdown Renders dropdowns
In Foundation 4, they wanted to rid their codebase of many different dropdowns within various UI elements. So they created a universal dropdown plugin that will attach dropdowns or popovers next to anything!
Example
Link Dropdown » Content Dropdown »Title
Some text that people will think is awesome! Some text that people will think is awesome! Some text that people will think is awesome!
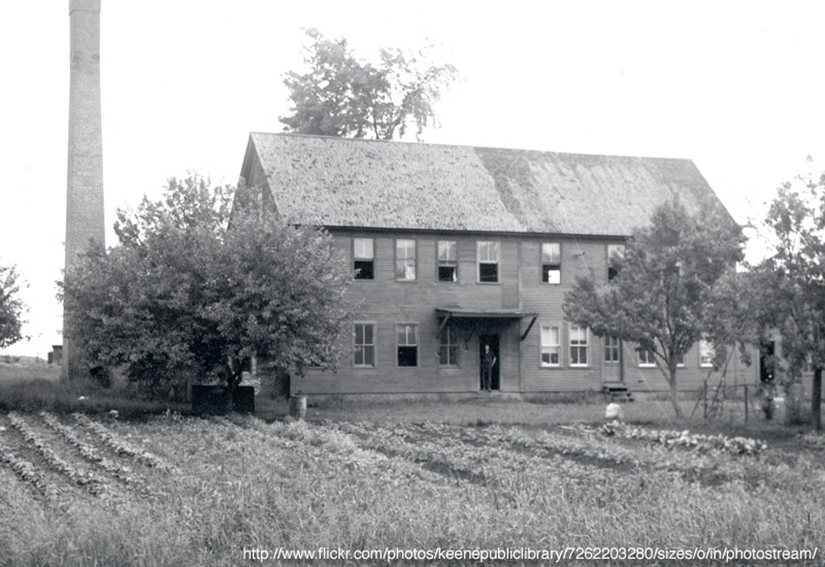
More test.
Button<?php echo Button::link('Link Dropdown »', array('data-dropdown' => 'tinyDrop'));?> <?php echo foundation\widgets\Dropdown::display(array( 'htmlOptions' => array('id' =>'tinyDrop'), 'items' => array( '<li>' . CHtml::link('This is a link') . '</li>', '<li>' . CHtml::link('This is another'). '</li>', '<li>' . CHtml::link('Yet another'). '</li>' ) ));?> <?php echo Button::link('Content Dropdown »', array('data-dropdown' => 'contentDrop'));?> <?php echo foundation\widgets\Dropdown::display(array( 'htmlOptions' => array('id' =>'contentDrop', 'class' => Enum::DIALOG_MEDIUM), 'type' => Enum::DROPDOWN_CONTENT, 'dropdownContent' => '<h4>Title</h4> <p> Some text that people will think is awesome! Some text that people will think is awesome! Some text that people will think is awesome! </p> <img src="/images/demos/demo1.jpg"> <p>More test.</p> <a href="#" class="button">Button</a>' ));?>
FlexVideo Embeds and scales videos
If you're embedding a video from YouTube, Vimeo, or another site that uses iframe, embed or object elements, you can wrap your video in div.flex-video to create an intrinsic ratio that will properly scale your video on any device.
Example
4:3 is the default size for the .flex-video element, and the assumption for .flex-video for chrome (controls) height is based on YouTube.
Because Vimeo places their chrome on the player itself, adding a class of .vimeo creates a container that is sized for the video only — no extra padding for the controls.
<div class="row"> <div class="large-6 columns"> <?php echo foundation\widgets\FlexVideo::display(array( 'source' => 'http://www.youtube.com/embed/0_EW8aNgKlA' )); ?> </div> <div class="large-6 columns"> <?php echo foundation\widgets\FlexVideo::display(array( 'source' => 'http://player.vimeo.com/video/21762736', 'vimeo' => true )); ?> </div> </div>
InterChange Load different media sizes
Interchange uses media queries to load the images that are appropriate for a user's browsers, making responsive images a snap.
Example
<?php echo foundation\widgets\Interchange::display( array( 'src' => 'http://foundation.zurb.com/docs/img/demos/interchange/med.jpg', 'rules' => array( array( 'http://foundation.zurb.com/docs/img/demos/interchange/smallest.jpg', '(default)' ), array( 'http://foundation.zurb.com/docs/img/demos/interchange/smallest.jpg', '(screen and (max-width: 568px))' ), array( 'http://foundation.zurb.com/docs/img/demos/interchange/small.jpg', '(small)' ), array( 'http://foundation.zurb.com/docs/img/demos/interchange/med.jpg', '(medium)' ), array( 'http://foundation.zurb.com/docs/img/demos/interchange/large.jpg', '(large)' ), ) ) ); ?>
JoyRide Touring made simple
Joyride is an extremely flexible plugin that gives users a tour of your site or app when they visit.
Heads up! In order to position the tour tip on different locations (top,
bottom, left, right), we should make use of the options
attribute of each stop configuration
and set the autoStart
property to true.
Example
First stop
This is the first stop
Second Stop
This is the content for second stop
Third Stop
This is the content for third
Finally at the end
This is the end
<?php echo foundation\widgets\JoyRide::display( array( 'autoStart' => false, 'stops' => array( array( 'id' => 'joyride-one', 'button' => 'Next', 'title' => 'First stop', 'body' => 'This is the first stop' ), array( 'id' => 'joyride-two', 'button' => 'Next', 'title' => 'Second Stop', 'body' => 'This is the content for second stop', ), array( 'id' => 'joyride-third', 'button' => 'Next', 'title' => 'Third Stop', 'body' => 'This is the content for third' ), array( 'button' => 'Finish', 'title' => 'Finally at the end', 'body' => 'This is the end' ) ) ) ); ?> <div> <span id="joyride-one">Here is the first stop</span> | <span id="joyride-two">Here is the second stop</span> | <span id="joyride-third">Here is the third stop</span> | <?php echo Button::link('Start Tour', array( 'onclick' => '$(document).foundation("joyride", "start");')); ?> </div>
Magellan Sticky navigations
Magellan is a style agnostic plugin that lets you style a sticky navigation that denotes where you are on the page.
We have configured Magellan at the top of this page. Whenever you scroll down the page, the Magellan plugin will active the correspondent item as it arrives to its target.
This is how we configured the plugin on this page:
<?php echo foundation\widgets\Magellan::display( array( 'items' => array( array('id' => 'alert', 'label' => 'Alert', ''), array('id' => 'breadcrumbs', 'label' => 'Breadcrumbs'), array('id' => 'clearing', 'label' => 'Clearing'), array('id' => 'dropdown', 'label' => 'Dropdown'), array('id' => 'flexvideo', 'label' => 'FlexVideo'), array('id' => 'interchange', 'label' => 'InterChange'), array('id' => 'joyride', 'label' => 'JoyRide'), array('id' => 'magellan', 'label' => 'Magellan'), array('id' => 'orbit', 'label' => 'Orbit'), array('id' => 'pager', 'label' => 'Pager'), array('id' => 'reveal', 'label' => 'Reveal'), array('id' => 'section', 'label' => 'Section'), array('id' => 'switchbutton', 'label' => 'SwitchButton'), array('id' => 'tooltip', 'label' => 'Tooltip'), array('id' => 'topbar', 'label' => 'TopBar'), ) ) ); ?>
As you can see above, the id
of each element is set to the destination element. The
destination element is configured as follows:
<a name="magellan"></a> <h3 data-magellan-destination="magellan">Magellan <small>Sticky navigations</small></h3>
Do you see that the id of the Magellan item is actually the same as the anchor element and the
data-magellan-destination
attribute? Easy stuff :)
Orbit Image slideshow
Orbit is an easy to use, powerful image slider that's responsive, allowing you to swipe on a touch-enabled device.
Example
<?php echo foundation\widgets\Orbit::display(array( 'htmlOptions' => array('style' => 'width:645px'), 'items' => array( array( 'content' => '<img src="/images/orbit-demo/demo1.jpg" alt="slide image">', 'caption' => 'Mi caption 1'), array( 'content' => '<img src="/images/orbit-demo/demo2.jpg" alt="slide image">', 'caption' => 'Mi caption 2'), array( 'content' => '<img src="/images/orbit-demo/demo3.jpg" alt="slide image">', 'caption' => 'Mi caption 3') ) )); ?>
Pager Handles pagination
Moving between pages has become less common with the advent of longer pages and AJAX loading, but it can still be useful for long, repetitive listings or content.
We have created a CLinkPager child to attach to your grids
Example
<?php $pagination = new CPagination(); $pagination->itemCount = 100; $pagination->pageVar = 'test_page'; $pagination->pageSize = 5; echo foundationwidgetsPager::display( array( 'pages' => $pagination ) ); ?>
Reveal Modals Foundation style
Modal dialogs, or pop-up windows, are handy for prototyping and production. Foundation includes Reveal our jQuery modal plugin, to make this easy for you.
Example
<?php echo foundation\widgets\Reveal::display( array( 'header' => 'This is simple modal', 'body' => 'This is my body modal', \\ you can use partial views 'events' => array( 'open' => 'js:function(){console.log("open");}', 'opened' => 'js:function(){console.log("opened");}', 'close' => 'js:function(){console.log("close");}', 'closed' => 'js:function(){console.log("closed");}' ), 'toggleButton' => array( 'label' => 'Click to Open' ) ) ); ?>
Section Another perspective of tabs
Sections are similar to tabs as a way to selectively show a single panel of content at a time.
Example
Auto Tabs
Accordion
Vertical Tabs
<?php echo foundation\widgets\Section::display( array( 'style' => Enum::SECTION_STYLE_VERTICAL_TABS, 'items' => array( array( 'label' => 'Section 1', 'content' => CHtml::tag('p', array(), 'Section 1 content') ), array( 'label' => 'Section 2', 'content' => CHtml::tag('p', array(), 'Section 2 content'), 'options' => array('id' => 'panchito'), 'active' => true ) ), ) ); ?>
SwitchButton Switching between two options
SwitchButtons can be used instead of regular radio buttons to switch between two options. They are customizable and use styles that won't show on phones that don't support media queries. On these devices, they will appear as regular radio buttons.
Example
<?php echo foundation\widgets\SwitchButton::display(array( 'name' => 'test-switch-1', 'size' => Enum::SIZE_LARGE, 'htmlOptions' => array('class' => 'small-6') )); ?> <?php echo foundationwidgetsSwitchButton::display(array( 'name' => 'test-switch-2', 'size' => Enum::SIZE_LARGE, 'radius' => Enum::RADIUS_RADIUS, 'htmlOptions' => array('class' => 'small-6') )); ?> <?php echo foundationwidgetsSwitchButton::display(array( 'name' => 'test-switch-3', 'size' => Enum::SIZE_LARGE, 'radius' => '', 'htmlOptions' => array('class' => 'small-6') )); ?>
Tooltips Render extended information tips
Tooltips are a quick way to provide extended information on a term or action on a page.
Example
hover me for bottom tooltip | This is pure HTML markup now
<?php echo foundation\widgets\Tooltip::display( array( 'id' => 'a', 'tip' => 'This is a cool tooltip', 'text' => 'hover me for bottom tooltip', 'position' => Enum::TOOLTIP_BOTTOM ) ); ?> | <span data-tooltip class="has-tip" title="Once the widget is used, we can use set up tooltips with HTML markup only!"> This is pure HTML markup now </span>
TopBar Navigation bar
The Foundation Top Bar gives you a great way to display a complex navigation bar on small or large screens.
Example
<?php echo foundation\widgets\TopBar::display( array( 'title' => '<h1><a href="#">YiiFoundation Rocks</a></h1>', 'rightItems' => array( array( 'label' => 'top item', 'url' => '#', 'items' => array( array('label' => 'dropdown 1', 'url' => '#') ) ) ) ) ); ?>